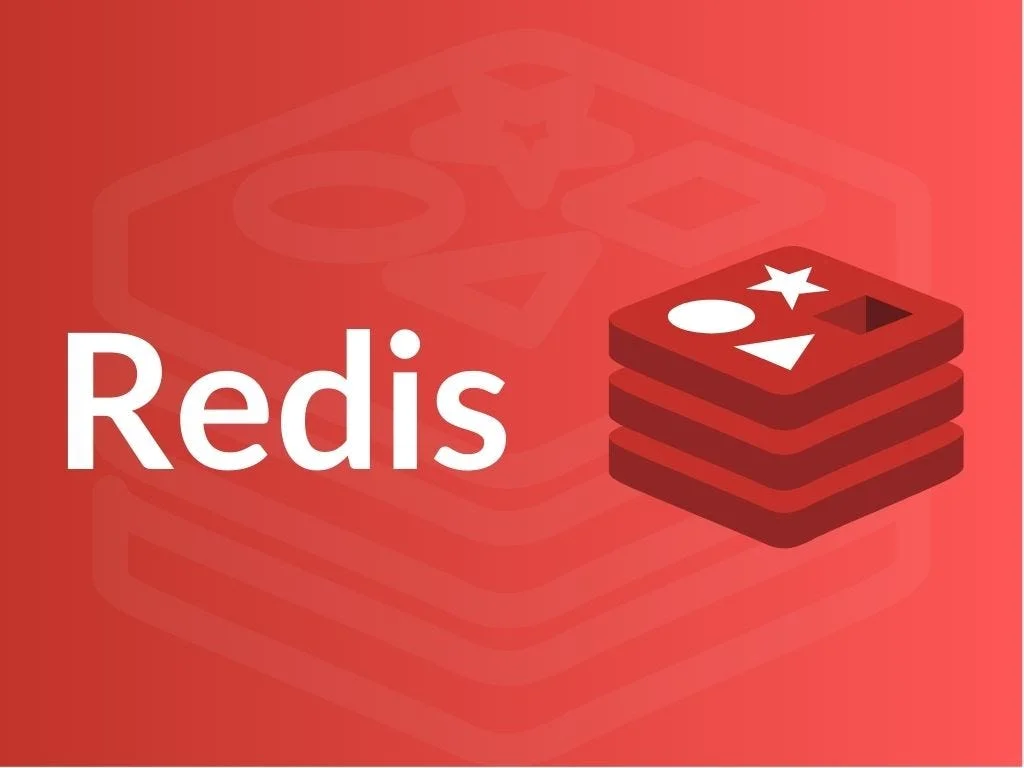
Redis is a high-performance in-memory data store known for speed and versatility. Yet, handling large values presents challenges, especially where memory is a limited resource. We’ll explore methods to efficiently manage large values in Redis without overburdening memory.
Why Large Values Pose a Challenge in Redis?
Redis stores data in memory for quick access, but large values can quickly deplete available resources, affecting performance and stability. This is critical for applications like log storage or caching, where balance between performance and memory usage is vital.
- Memory Limits: Large data can exhaust available memory, affecting systems with limited RAM.
- Fragmentation: Big data blocks may cause inefficient use of memory due to fragmentation.
- Durability: Managing persistence while handling large values can introduce complexity.
What is Considered a Large Value in Redis?
Values exceeding 10KB in Redis are typically seen as large. This benchmark can shift based on total memory, number of keys, access patterns, and the specific needs of your application.
Examples of Large Values:
- JSON Document: Detailed records like orders or user data, ranging 50KB to 500KB.
- Image Data: Base64 encoded images, typically 100KB-2MB for medium resolution.
- Session Data: User sessions with large arrays or logs, ranging 20KB-100KB.
- Log Entries: Intensive logging data up to 200KB per entry.
- Cached API Response: Detailed product catalogs or similar data, often 100KB-1MB.
Strategies to Manage Large Values in Redis
(i) Splitting Data into Smaller Chunks
Segregating a large value into manageable chunks facilitates better memory management and minimizes fragmentation risks.
// Split and store data
SET log:123:chunk:1 "chunk data 1"
SET log:123:chunk:2 "chunk data 2"
// Metadata for chunks
HSET log:123:metadata total_chunks 2
// Retrieve chunks
HGET log:123:metadata
GET log:123:chunk:1
GET log:123:chunk:2
# Example in Python
import json
class RedisChunker:
def __init__(self, redis_client, chunk_size=1024*1024): # 1MB chunks
self.redis = redis_client
self.chunk_size = chunk_size
def store_chunked(self, key, data):
chunks = [data[i:i + self.chunk_size] for i in range(0, len(data), self.chunk_size)]
self.redis.hset(f"{key}:metadata", mapping={"total_chunks": len(chunks), "size": len(data)})
for i, chunk in enumerate(chunks, 1):
self.redis.set(f"{key}:chunk:{i}", chunk)
def retrieve_chunked(self, key):
metadata = self.redis.hgetall(f"{key}:metadata")
if not metadata:
return None
return b''.join(self.redis.get(f"{key}:chunk:{i}") for i in range(1, int(metadata[b'total_chunks']) + 1))
chunker = RedisChunker(redis_client)
large_data = "..." * 1024 * 1024 # Large string
chunker.store_chunked("my_large_value", large_data.encode())
(ii) Using Compression
Apply compression algorithms like zlib before storing large values to significantly conserve memory resources.
import zlib
# Compress before storing
compressed_value = zlib.compress(large_value.encode())
redis_client.set("log:123", compressed_value)
# Decompress when retrieving
compressed_value = redis_client.get("log:123")
original_value = zlib.decompress(compressed_value).decode()
(iii) Utilizing Redis Streams
Use Redis Streams for high-throughput, log-like data management. Streams handle data neatly and offer automatic trimming options.
// Add log entry
XADD logs MAXLEN ~10000 * message "Log message here"
// Read logs
XRANGE logs - +
# Example in Python
from datetime import datetime
import json
class RedisStreamManager:
def __init__(self, redis_client, stream_name, max_len=10000):
self.redis = redis_client
self.stream = stream_name
self.max_len = max_len
def add_entry(self, data: dict):
data['timestamp'] = data.get('timestamp', datetime.utcnow().isoformat())
entry = {k: json.dumps(v) if isinstance(v, (dict, list)) else str(v) for k, v in data.items()}
return self.redis.xadd(self.stream, entry, maxlen=self.max_len, approximate=True)
def read_range(self, start='-', end='+', count=None):
entries = self.redis.xrange(self.stream, start, end, count)
return [
(entry_id.decode(), {k.decode(): json.loads(v.decode()) if isinstance(v, bytes) else v.decode() for k, v in entry_data.items()})
for entry_id, entry_data in entries
]
stream_manager = RedisStreamManager(redis_client, "app_logs")
stream_manager.add_entry({"level": "INFO", "message": "User login", "metadata": {"user_id": 123, "ip": "192.168.1.1"}})
(iv) Offloading Large Data to External Storage
For infrequently accessed large values, use Redis for metadata while storing the actual data in external systems like databases or cloud storage.
HSET log:123 metadata '{"size": "100MB", "location": "s3://mybucket/log-123"}'
(v) Using Binary Encoding
Binary formats such as Protocol Buffers or MessagePack can effectively reduce data size in Redis.
(vi) Enabling Disk Persistence
Leverage Redis persistence modes for data durability, with RDB for snapshots and AOF for logging every write operation.
save 60 10000 // RDB: Every 60 seconds if 10,000 keys are changed
appendonly yes
appendfsync everysec
(vii) Using a Redis Cluster
Deploying a Redis Cluster distributes keys across nodes, increasing memory capacity without singular node overload.
(viii) Setting Key Expiration
Automatically remove outdated entries by setting expiration times, essential for logs or temporary caches.
SET log:123 "large value" EX 3600 // Expires in 1 hour
(ix) Monitoring Memory Usage
Ongoing memory monitoring helps identify inefficiencies, allowing for swift adjustments and optimization.
MEMORY USAGE key_name
INFO memory
(x) Optimizing Memory Settings
Configure Redis to prevent memory exhaustion and maintain smooth operations.
// Compact memory
MEMORY PURGE
// Set memory limits to prevent overconsumption
maxmemory 1.5gb
maxmemory-policy noeviction
By applying these methods, you can manage large values in Redis optimally, maintaining performance and reliability across your applications. Routine monitoring and configuration fine-tuning can further enhance Redis’s capability to handle extensive data volumes effectively.