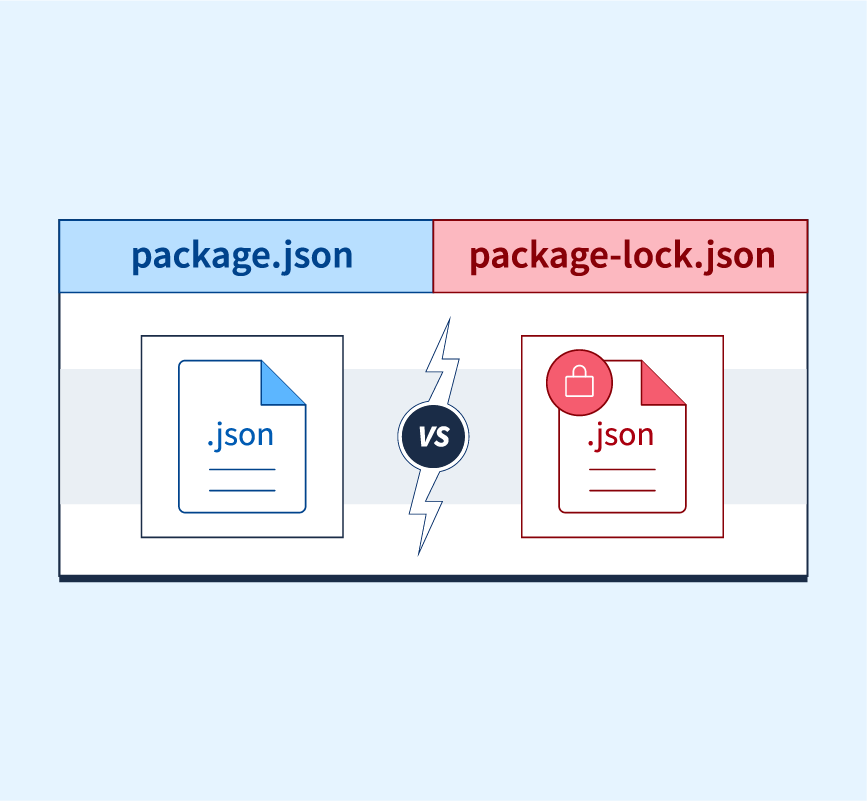
In the world of Node.js, efficient dependency management underpins a project’s success. Two vital components in this process are package.json
and package-lock.json
. Both offer critical information and functionality, but serve different purposes in maintaining your project’s coherence. Let’s explore each file’s role and how they work together.
What is package.json?
The package.json
file is the cornerstone of any Node.js project, acting as a manifest that describes the project’s metadata, dependencies, scripts, and configuration details. It specifies essential information like the project name, version, author, and license, as well as which external packages or modules the project requires to function correctly. This file allows developers to streamline setup and collaboration by providing a standardized way to manage these dependencies and configurations.
Example snippet:
{
"name": "project-name",
"version": "1.0.0",
"description": "A description of the project",
"main": "index.js",
"scripts": {
"start": "node index.js"
},
"author": "Jane Doe",
"license": "MIT",
"dependencies": {
"express": "^4.16.4"
}
}
The Purpose of package.json
- Dependency Management: Specifies required dependencies and their version ranges.
- Metadata: Describes the project’s details for easy identification.
- Scripts and Configuration: Defines scripts for build, test, start, and other operations.
- Publishing: Provides information necessary for publishing the project to registries like npm.
Tilde (~) and Caret (^) Notations
These symbols in versioning help control updates:
- Tilde (~): Updates patch versions but maintains the current major and minor version.
- Caret (^): Allows updates for minor versions and patches, offering a broader range of updates.
What is package-lock.json?
The package-lock.json
file captures the exact resolved versions of dependencies at the time of installation. This ensures that everyone using the project gets the consistent experience across different environments, crucial for avoiding conflicts and ensuring compatibility.
Example
This is what a package-lock.json file looks like –
Purpose of package-lock.json
- Consistency: Locks down specific versions of each package.
- Reliability: Facilitates reproducible builds and faster installations.
- Security: Maintains verified and secure dependencies.
- Reproducibility: Ensures projects can be reliably built in any environment.
Comparing package.json with package-lock.json
Feature | package.json | package-lock.json |
---|---|---|
Role | Metadata and dependency management file | Dependency lock file for exact versions |
Content Maintenance | Manually maintained | Automatically generated |
Version Ranges | Utilizes semantic versioning (^,~) | Specifies exact installed versions |
Manual Edits | Yes, editable by developers | No, should not be manually edited |
Role in Version Control | Essential for information sharing | Ensures consistent builds across teams |
Focus | General project setup | Locked dependency tree |
What is npm-shrinkwrap.json?
The npm-shrinkwrap.json
file serves a purpose similar to package-lock.json
, ensuring exact dependency versions are used in production. However, unlike package-lock.json
, it’s used for projects where package versions must be consistent during distribution and when package-lock.json
is insufficient.
Use the command npm shrinkwrap
to create or update the shrinkwrap file.
Conclusion
Each of these files plays a unique role in maintaining a Node.js project. By using package.json
, package-lock.json
, and npm-shrinkwrap.json
adeptly, developers can manage dependencies effectively, ensuring that projects are built consistently and reliably across diverse environments.